WooCommerce will send the store administrator an email when a product’s inventory goes below a certain threshold. There are two global inventory thresholds used for all products. These thresholds are named “Low stock” and “Out of stock“.
The global setting works great for most stores, but for some it doesn’t work as well as it could. In this post I will show you how to set up a WooCommerce Low Stock Notification per product and category.
As an example we will use a hardware store selling tools and home improvement products. A global low stock threshold of 5 works when a product like an electric drill gets low on stock, but if a product like screws falls below 5 in stock that number is far too low. For a product like screws you’d like to have at least 500 in stock, so this requires a different low stock notification threshold.
Setting Up a Low Stock Notification
In this post, I’ll share a few code snippets that will help you set up low stock notification for an entire WooCommerce category or an individual product.
Note: If you use one of the code snippets found below know that:
- These code snippets work with the latest version of WooCommerce.
- It is a good idea to disable the default low stock notifications otherwise products might trigger both the default and custom setup notification.
- If you are not sure how to add these code snippets to your site we described how to add custom snippets in this blog post.
Low stock notification per category
Setting up a low stock notification per category can make sense in a lot of cases such as the example above. Setting up a separate low stock threshold per product may take more effort than needed in some scenarios.
The following code snippet can be used to set up a different low stock notification per category. You can modify your category slugs and threshold in the code. If you want to add more custom thresholds, just add a new line in the array and set the category-slug threshold.
/**
* Send custom low stock notifications per category
*
* @param WC_Order $order
*/
function sp_custom_low_stock_notification_category( $order ) {
/**
* Set a different threshold per category-slug.
* The category slug is set in the 'key' and the stock threshold the 'value'.
*/
$stock_thresholds_category = array(
'drills' => 5,
'screws' => 500,
'paint' => 10,
);
foreach ( $order->get_items() as $item ) {
if ( $item->is_type( 'line_item' ) && $product->managing_stock() ) {
$product = $item->get_product();
$new_stock = $item->get_quantity();
// Loop through stock threshold categories
foreach ( $stock_thresholds_category as $cat => $threshold ) {
// If the product has the stock categories AND is below the threshold, send notification
if ( has_term( $cat, 'product_cat', $product->get_id() ) && $new_stock < $threshold ) {
do_action( 'woocommerce_low_stock', $product );
}
}
}
}
}
add_action( 'woocommerce_reduce_order_stock', 'sp_custom_low_stock_notification_category', 10 );
Improving the Low Stock Notification Per Product
In some cases it makes sense to have a low stock notification per product. WooCommerce now includes a Low stock threshold field in the core plugin. This field can be viewed by going to the Edit Product page, then click on the Inventory tab.
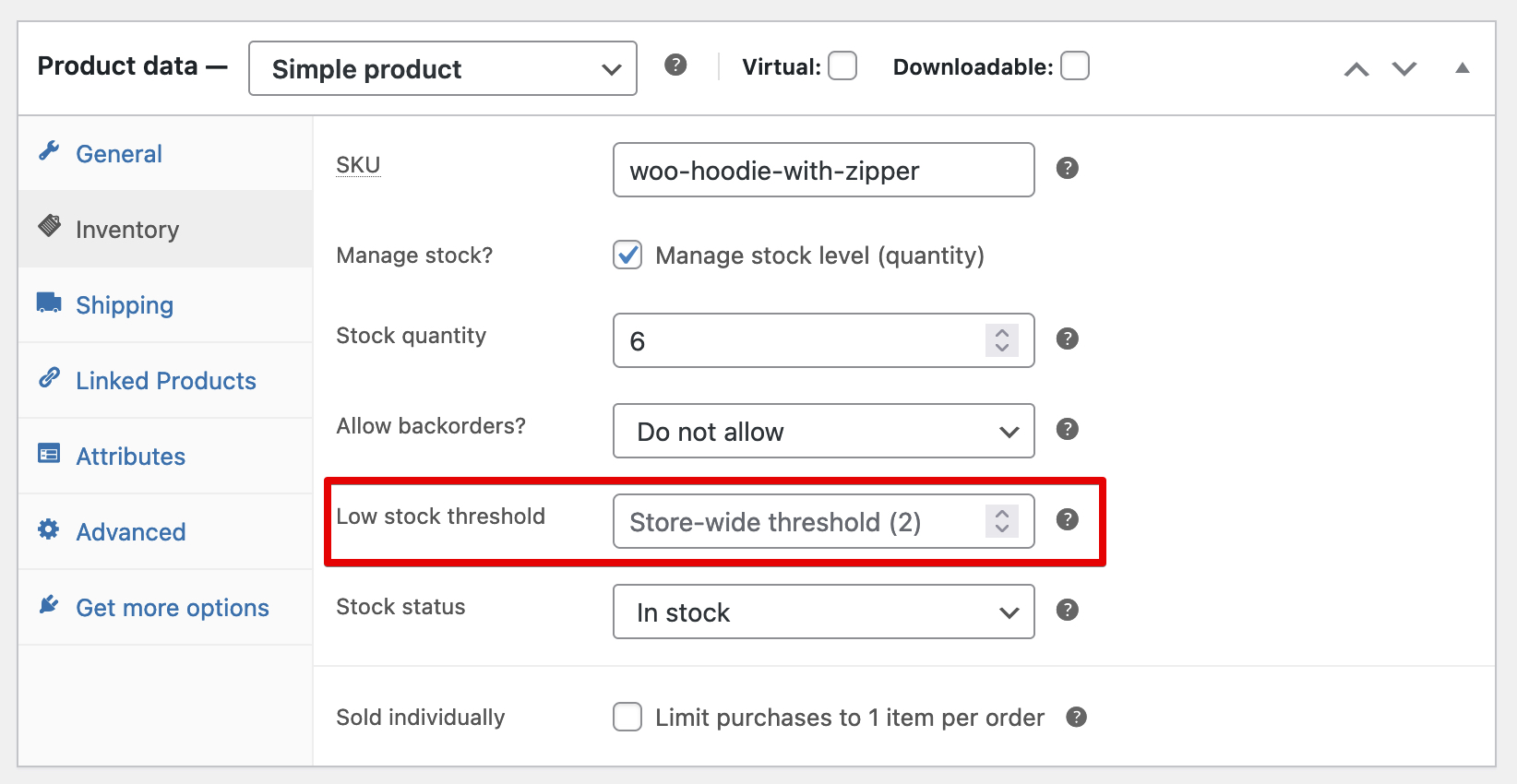
The setting can be updated for each individual product, and also can be set on variations.
Low Stock Notification Per Product Programmatically
The following code snippet is similar to the one for categories, but instead uses a product ID to set the threshold.
/**
* Send custom low stock notifications per product
*
* @param WC_Order $order
*/
function sp_custom_low_stock_notification_product( $order ) {
/**
* Set a different threshold per product ID.
* The Product ID is set in the 'key' and the stock threshold the 'value'.
*/
$stock_thresholds_products = array(
47 => 10,
24 => 7,
26 => 3,
);
foreach ( $order->get_items() as $item ) {
if ( $item->is_type( 'line_item' ) && $product->managing_stock() ) {
$product = $item->get_product();
$new_stock = $item->get_quantity();
// Loop through stock threshold products
foreach ( $stock_thresholds_products as $product_id => $threshold ) {
if ( $product->get_id() == $product_id && $new_stock < $threshold ) {
do_action( 'woocommerce_low_stock', $product );
}
}
}
}
}
add_action( 'woocommerce_reduce_order_stock', 'sp_custom_low_stock_notification_product', 10 );
This snippet can be useful if you the inventory notification thresholds change often and it’s not possible to update several thousand products via the WooCommerce dashboard. The array in the snippet can be updated easily and quickly.
Wrapping Up
If you decide adding your own custom code is too difficult, I recommend checking out our WooCommerce Advanced Messages Plugin. It will allow you to create low stock notices for any product based on conditional settings, without having to modify any code. These conditions are easy to setup and easy to maintain.
Happy Selling!
Keep up-to-date with the weekly newsletter
You will receive a bonus tips, actionable insight, and plugin updates straight to your inbox!